Shortly after downloading and installing Apple’s new Mac OS X Lion this morning, I started compiling a list of what I liked and what I did not like. My excitement began to fade as I realized there was a lot I was not going to enjoy about Apple’s new OS. After spending the morning with OS X Lion, I present a review that highlights both the good and bad of the new OS, along with tips to revert some new, possibly unwelcome features.
The Good
1) Launchpad: Launchpad is a new user interface component, which allows you to view the Applications on your computer in an iOS-like fashion. While I am not sure I will use this new interface, I like that Apple is trying to bridge the Desktop OS X and Mobile iOS worlds with a more consistent interface.
|
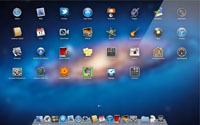 |
2) Mission Control: Although this feature is a bit recycled from Exposé, I do enjoy the new look and organization of the application overview.
|
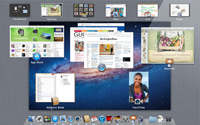 |
3) Easy Access to DVD Media: When OS X Lion was first announced, there was a lot of uproar about the digital-only release. There were many who worried about how to do a fresh install of Lion on a new hard drive and the instruction, purportedly from Steve Jobs, that they install an old version of Mac OS then upgrade to Lion was met with a lot of online criticism. Most of those issues have since disappeared as Apple has provided a method, possibly unsupported, for creating a DVD image of the downloaded Lion installation. The steps are extremely easy and I had the DVD created within minutes of downloading. I tested the DVD on another Mac in my household, as the OS X license permits up to 5 installations, and the DVD worked without issues.
4) Full-screen Applications: The ability to load applications in full-screen was first introduced in iLife about a year ago and has now been extended throughout the OS. As someone who values screen real estate, the new feature is a welcome addition, especially if you are using an 11″ MacBook Air.
5) New Coat of Paint: The overall graphical interface for Lion is a lot more crisp and appealing, succeeding with that ‘lickable’ aspect Apple is known for.
6) Java Support: Like the DVD media issue, there were a lot of rumors predicting the end of Java on OS X Lion that have turned out to be false. Although there is some evidence to suggest Apple will be taking a backseat to integrating Java with the OS in the future, they have already posted a Java 1.6 release available as a separate download. They have also added an automatic download feature that detects when Java is required and automatically installs it. For example, the first time I started Eclipse, Java 1.6.0_26 (64-bit) was retrieved and installed within seconds. In short, Java is still very much alive and well in OS X Lion despite rumors to the contrary.
The Bad
1) Microsoft Office 2004 Dead: OS X Lion removes Rosetta compatibility, which means older applications such as Word, Excel, or PowerPoint 2004 will no longer load. In fact, Lion highlights such applications as shown in this screenshot. I am actually a big fan of Office 2004, as I have used more recent versions and been turned off by the annoying ribbon interface. Office 2004 was one of the best versions of Office and is now completely dead in Lion.
|
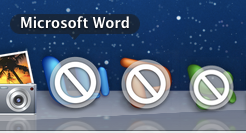 |
2) Slow: Ever since I started is up, OS X Lion has had an unreasonably slow user interface, especially in terms of responsiveness. As early as the login screen, I had to wait 10+ seconds after selecting my username to enter my password. My computer may not be fresh off the assembly line but it is still a somewhat new high-end MacBook Pro, so I’m shocked at the user interface delay in loading and using programs. Even loading System Preferences and the OS X Updater took a lot of time.
The reason for these delays may be that Spotlight runs a re-index of the hard-drive immediately following installation, but I’ll have to wait until it is finished to know for sure. Initially, Spotlight reported “4 weeks remaining” but that has since changed to 7 hours, which is better, but still a lot of time to wait. The extra process is also causing the temperature to spike and it practically burns my hand to type right now. It’s like taking a new sports car out on the road but not being able to go above 5 miles per hour, while the seat slowly burns your legs. It certainly diminishes the initial user experience after installation and should have been scheduled to occur at a later time.
Update [7/22/2011]: After Spotlight finished re-indexing the hard-drive and Time Machine ran its multi-gigabyte backup, the computer is running much better again. I still notice some impact lag when typing passwords and launching certain applications, but I can’t say for sure if this was much different than before I upgraded. In retrospect, I still contend Spotlight should have postponed its re-index of the hard-drive as this created a poor user experience immediately following installation.
3) Unnatural scrolling: For some reason, Apple decided to reverse the scroll direction. Up is down, down is up, left is right, etc. According to the documentation is this a more ‘natural’ approach, but from a user interface perspective reversing such a now-key part of the user interface is ridiculous. Luckily there is a way to disable this ‘feature’. Open System Preferences, select Trackpad, and uncheck the option for Scroll direction: natural. On older Macs, Apple presents this in a different manner, and to disable it, uncheck When using gestures to scroll or navigate, move content in the direction of finger movement
4) Launchpad + Parallels: One area where I found this particularly troublesome is if you have Parallels installed, it lists dozens, or even hundreds, of Parallels applications in Launchpad, often outweighing the number of OS X applications. This may not bother a lot of people, especially those that keep Parallels open at all times, but was extremely frustrating for myself. These items can be removed from Launchpad by opening Parallels and selecting the virtual machine. Then from the menu bar then select Virtual Machine, click Configure, select Applications, then uncheck Share Windows applications with Mac.
5) iChat Single View: iChat has been updated to automatically merge multiple buddy lists into one. Some people may like this feature, but I found annoying that it was enabled by default. Lion also overwrote many of my default preferences, such as hiding the hundreds of Offline Buddies on my list. None of these are killer issues, but did annoy me the first time I loaded iChat. To disable it, select Preferences from the menu bar, click General, and uncheck Show all my accounts in one list.
6) iChat won’t save account passwords: I’m having an issue that every time I start iChat I need to enter passwords for each of my accounts, despite having previously saved them in my keychain before the upgrade as well as checking the box to save them in my keychain now. I haven’t heard anyone else report this issue yet, but something seems buggy here.
Update [7/22/2011]: One website suggested my iChat preferences had been corrupted. The fix is to navigate to ‘/Users//Library/Preferences’ and delete all com.apple.iChat* files. You must then log out for the fix to work and re-setup iChat the next time you log in. I’m pleased to report this solution does work and I can now open and close iChat without having to reenter my passwords each time it starts up. Has anyone else installed OS X Lion, only to have their iChat preferences corrupted?
Some users have reported similar issues with Calendar passwords after upgrading to OS X Lion in which the solution was also to manually delete user Library files.
7) Adobe CS2 Dead: As Adobe has pointed out CS2 and earlier versions of its products do not function in OS X Lion due to the same Rosetta issue that disabled Microsoft Office 2004. More recent versions, such as CS3/CS4/CS5 still have a number of issues as Adobe spells out in its release notes.
Some components of Photoshop, such as Droplets, the automation creation tool, were sloppily implemented in PowerPC even in recent Intel-based versions of Photoshop. Adobe has released an update for CS5, although it is not clear if they will be updating CS3 or CS4, but given Adobe’s update history for older versions of it’s product, it seems unlikely.
Conclusion
Overall, I’m about 35% happy with OS X Lion. While I like the streamlined interface, new Mail client, and increased feature set, I can’t help but think they needed to spend more time on this. The erasing of many of my existing settings throughout the OS and applications, as well as needing to wait hours for the computer to finish running Spotlight before I can use it without burning my hands, leaves a bitter taste in my mouth. The biggest showstopping feature is the lack of support for Microsoft Office 2004, as I consider this version to be superior to many of the newer versions. More of my impressions will follow as I get more familiar with the many new features of this OS.