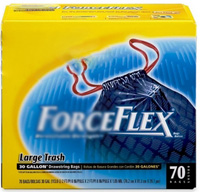 |
Did you know defining an event handler on an object in Flex can prevent it or other objects from being garbage collected even after all explicit references to the objects have been removed? If not, then keep reading. |
Introduction
Previously, I discussed techniques for freeing up memory on video and images in Flex, but I neglected to include a discussion of event handlers. With the release of ActionScript 3, Adobe added the notion of strong references and weak references. Strong references are those references that, if present, will prevent the object from being garbage collected. The presence of only weak references, on the other hand, will allow an object to be garbage collected. When most developers consider garbage collection, they often consider only strong references, those references they explicitly know about. The age-old wisdom, that if you remove all active (strong) references to an object, it will become eligible for garbage collection, overlooks the fact that the object may have strong references from other objects, created via event handler registration.
Event Handlers are Strong References (by default)
Somewhat surprisingly, and perhaps in a move to preserve older source code, the commonly used method addEventListener() creates a strong reference to the event handler by default. That means even after removing all explicit references to an object, it may never be eligible for garbage collection because other objects have subscribed as listeners to the object. If you have a process that repeatedly creates such objects, you have an uncontrolled memory leak that will eventually crash your program. As an example, consider a screen saver application that displays a new Image or Video on the screen every 30 seconds by creating new display objects and discarding the existing ones. Without considering the event handlers on this application, the memory usage could grow without bound, even if you take proper steps to discard the display objects.
From the Adobe Flex 3 documentation:
One important consequence of this parameter involves working with display objects’ events. Normally, you might expect a display object to be removed from memory when it is removed from the display list. However, if other objects have subscribed as listeners to that display object, with the useWeakReference parameter set to false (the default), the display object will continue to exist in Flash Player’s or AIR’s memory even though it no longer appears on the screen.
Solution #1: Proper deconstructor
The most obvious solution to prevent event handlers from holding objects in memory is to call removeEventListener() on the original object for each event handler on it. The problem is Flex does not provide a listAllEventListeners() method nor a removeAllEventListeners() method in the Event Dispatcher API [Feature Request!], so unless you remembered to save a reference to the event handler when you created it, you’re not going to have much luck removing it. This solution requires you to manage a list of event handlers and their associated types, so each can be discarded at a later time. Also, remember to remove the reference to the event handler in the list, such that the act of maintaining a list of references does not prevent garbage collection.
Solution #2: Declare a weak reference
Maintaining a list of event handlers for every object is a bit cumbersome, so luckily Adobe has added a better solution: declare the reference weak when the event is added to the object. In ActionScript 3, Adobe added an overloaded version of addEventListener() that takes 5 parameters. By setting the 5th parameter (useWeakReference) to be true, the event handler and its associated objects will become eligible for garbage collection if the weak reference event handlers are the only ones left.
For example, let’s say you defined a timer to run for 10 seconds and then stop:
var myTimer:Timer = new Timer(10,0);
myTimer.addEventListener(TimerEvent.TIMER,myTimerHandler);
As the code is written, objects referenced by myTimerHandler cannot be garbage collected, even if the timer was never started. Alternatively, if you add the overloaded parameters to the method ,false,0,true, objects referenced by myTimerHandler can be garbage collected:
var myTimer:Timer = new Timer(10,0);
myTimer.addEventListener(TimerEvent.TIMER,myTimerHandler,false,0,true);
Keep in mind, there are situations when it makes sense to define event handlers using strong references even on temporary objects. Just remember to explicitly remove the event handler if you want to reclaim the allocated memory.
Some developers, though, have made cases that Solution #1 is the only correct solution, and that using weak references is not sufficient for proper memory management.
Conclusion: Refactor your code with overloaded parameters
My suggestion to the reader is search through their code for all occurrences of addEventListener. For each occurrence, decide whether the event handler should live for the life of the application and never be garbage collected, or can be removed before the application completes. If it is the former, and the event handler should live indefinitely for the application, then do nothing. If it is the latter, and the event handler may be discarded at some point, add the parameters ,false,0,true to the event handler creation. This will preserve the existing functionality but allow it to be garbage collected. Keep in mind, though, that the event handler will not be garbage collected if you maintain strong or explicit references to it elsewhere. In the previous example, if you defined myTimerHandler to be a class-level member function, then the event handler would not be eligible for garbage collection with or without the change to the event handler registration.