Since the last time I took an exam, Oracle switched from Pearson VUE in person testing to online testing from your home/office. This series of three blog posts explains the process of buying a voucher and scheduling an exam. Some of the steps aren’t obvious, so I comment on those in more detail.
Remember to study before you buy the exam as your exam must be taken within 6 months of paying. See our Java 21 OCP Certified Professional Study Guide page for details!
- Part 1: Paying for the exam (you are here)
- Part 2: Assigning the exam attempt
- Part 3: Scheduling the exam
Step 1: Find the page for your exam
To start out, you find the page for your exam. For example, the Java SE 21 Developer Professional Exam Number: 1Z0-830. Alternatively, you can start from the page of all the exams.
Once on the page for your exam, note that there are three “steps”.
- “Recommended Training” – taking Oracle’s training is optional. It is also expensive so I recommend opting not to take it. Our Java OCP 21 Certified Professional Study Guide is two orders of magnitude less expensive.
- “Review Exam Topics” – this lists the exam objectives
- “Register and take the exam” – this section has a link to “Buy Exam”. There is also a link to “Buy Exam” at the top of the page before you get to these three steps. Both buttons take you to the same place so click either of them.
Step 2: Choose the Exam Type
The previous step takes you to a generic page for buying an exam with no memory of which exam it is. Choose the first option “Oracle Cloud Infrastructure and Technology Exams”. The Java Professional Cert is considered a “Technology Exam”. If you are not in the United States, you’ll see different prices. Click “Purchase” under that first column.
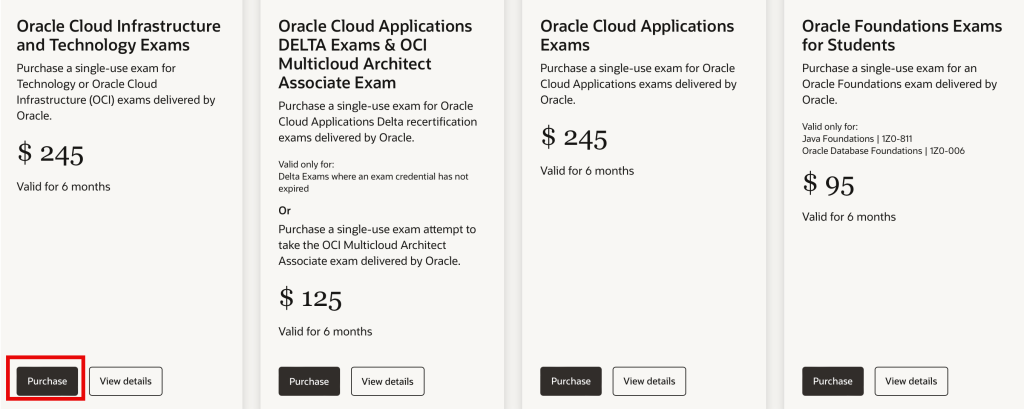
Note: If you are taking the Foundations exam click the fourth option. Unless you were specifically asked to, it is unlikely you are taking the Foundations exam. I wrote a blog post about why to choose the OCP instead back in Java 11 and the reasons still apply.
Step 3: Add to cart
You then get taken to a page inviting you to add a “subscription” to your cart. This page confirms Java is a choice for this type of certification.
Tip: the word “subscription” is misleading. It’s not a subscription. It’s a single attempt at taking one exam. It’s really an exam voucher which is not the same thing as a subscription. I guess they are sharing the processing engine with the Oracle University subscription option.
Choose “Add to Cart.”
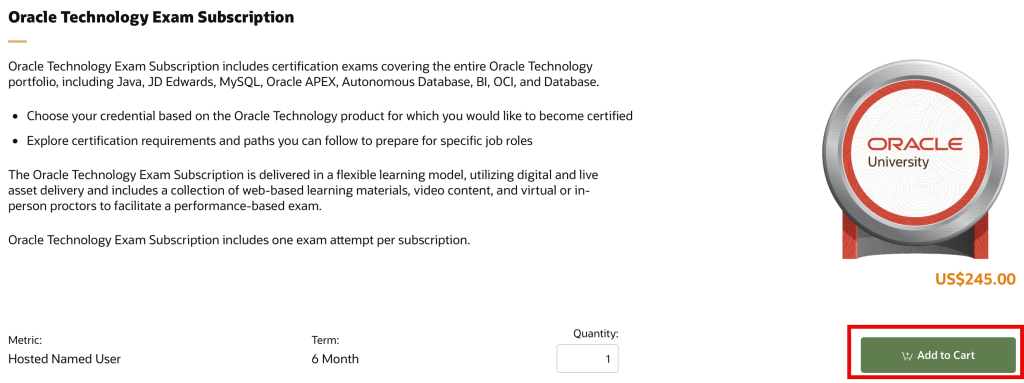
Step 4: Go to cart
After clicking “Add to Cart”, the page doesn’t take you to your cart. You need to click on the shopping cart in the top right corner manually which will give you a preview of your cart. Then click “View Cart” to actually go to your cart.
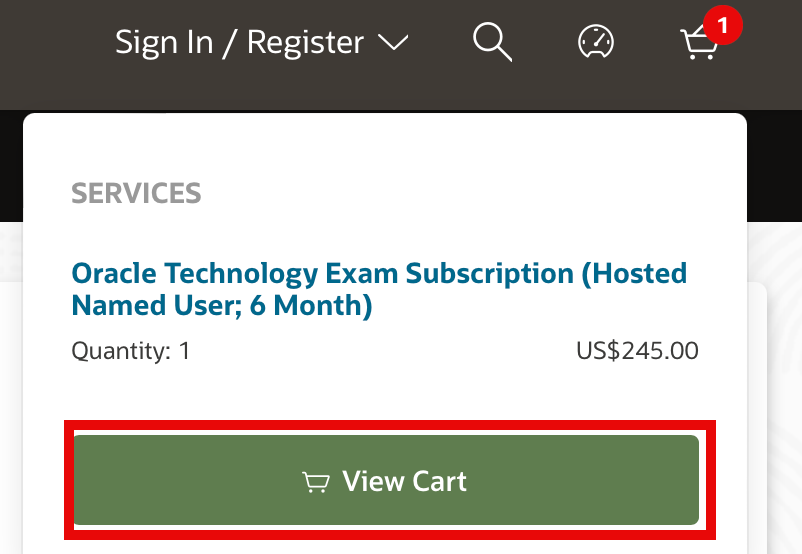
Step 5: Sign in (if you haven’t already)
You can sign in at any point in the process. When viewing the cart is the latest opportunity though.
Step 6: Checkout
Now you can click the “Checkout” button
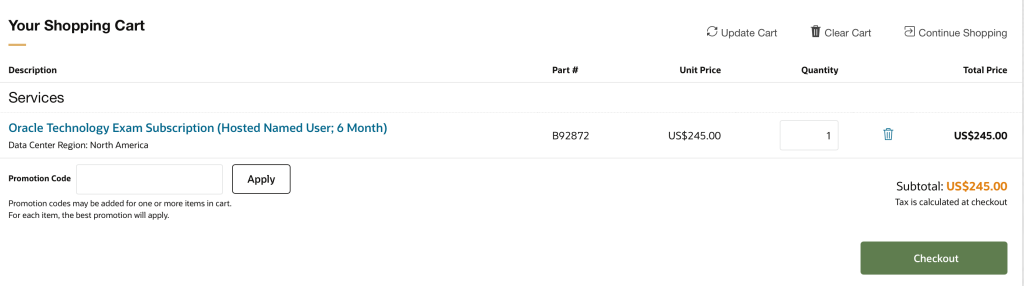
I’m not showing the checkout screen as it has my personal info on it, but they want to know
- Customer type – I choose Individual (there’s also an option for corporate). For individual, they want a contact phone number
- “Service” address. (aka mailing address) They wanted a county and town. Which is odd. The post office doesn’t want both in the United States
- Name/Email
- Phone number (yes, again)
- I clicked “preferred” for this address which defaulted the billing address to the same
- Payment info – added my credit card. Depending on where you live there may be tax. For Jeanne it was about $22 in tax
Then I clicked the “Place Order” button. I got taken to a screen with an order number and customer support id. it also says “Within 2 business days, you will receive an email from Oracle University containing a link and instructions to activate your subscription. If you have questions or need assistance, please Contact Us by filling out a support form under “Order Processing”.
Spoiler: it does not take two days. See Assigning the exam attempt for what to do when you get the exam.